This article uses the DHT22 and DHT11 temperature and humidity measurer modules with Python. They are sensor modules that operate using a single signal cable which will save the microcontroller’s port connection. In addition, MicroPython has a library prepared for DHT22 and DHT11 implementation, making it convenient, time-saving and reduce errors that may be caused by manual programming.
There are 3 examples in this article: code17-1 is a normal reading of values, but code17-2 is a loop to read again. By finding the highest and lowest values and display on the LCD module as shown in Figure 8 and example code17-3 showing the average value of temperature and humidity.
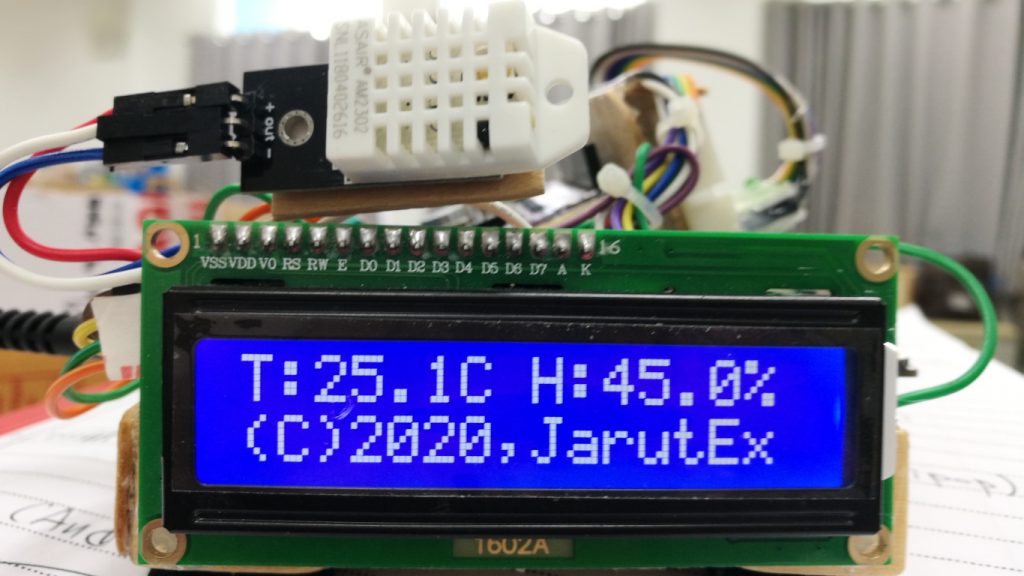
Modules
- ESP8266
- DHT11 or DHT22 In this article, we use the ready-made DHT22 module.
- I2C LCD 16×2
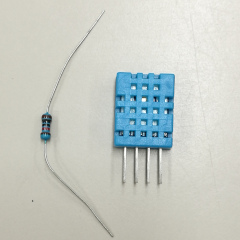
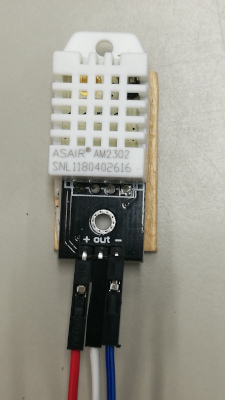
DHT22 and DHT11
The DHT22 and DHT11 modules are sensor modules that work with a single signal pin and uses a DC voltage of 3V3 to 5V5. The IC of DHT22 is AM2302. The differences between DHT22 and DHT11 are as follows.
Properties | DHT11 | DHT22 |
---|---|---|
Lowest temperature (C) | 0 | -40 |
Highest temperature (C) | 50 | 80 |
Temperature resolution (C) | +/- 2 | +/-0.5 – 1 |
Lowest humidity | 20% | 0% |
Highest humidity | 95% | 99.9% |
Humidity resolution | +/- 5% | +/- 2% |
Sampling Period (millisecond) | 1 | 2 |
The finished DHT22 module is as shown in Figure 3. It has the advantage of not having to connect the R-Pull up circuit and leaving only 3 connection pins for + or Vcc, – or GND and S for the data receiving/sending pin.
Connection
Connection between DHT22 sensor module and ESP8266
ESP8266 | DHT22 |
---|---|
5V | + |
GPIO2 (D4) | Sig |
GND | – |
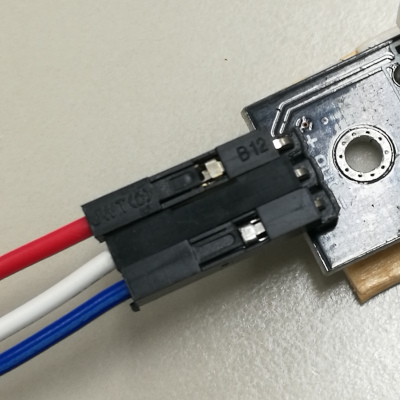
Connection between LCD module and ESP8266
ESP8266 | I2C LCD 16×2 |
---|---|
5V | Vcc |
GND | GND |
GPIO4 (D2) | SCL |
GPIO5 (D1) | SDA |
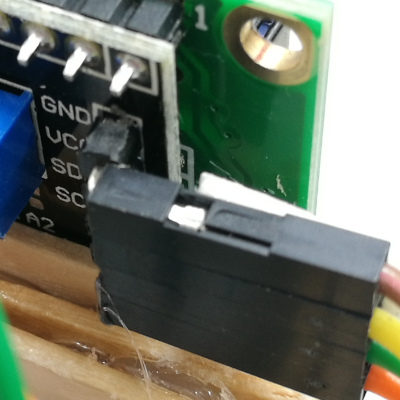
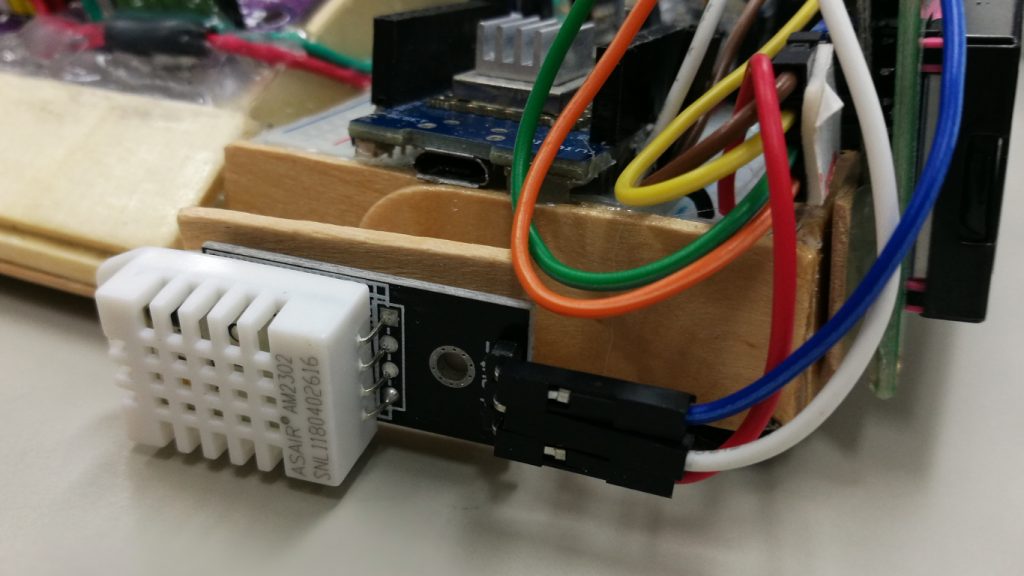
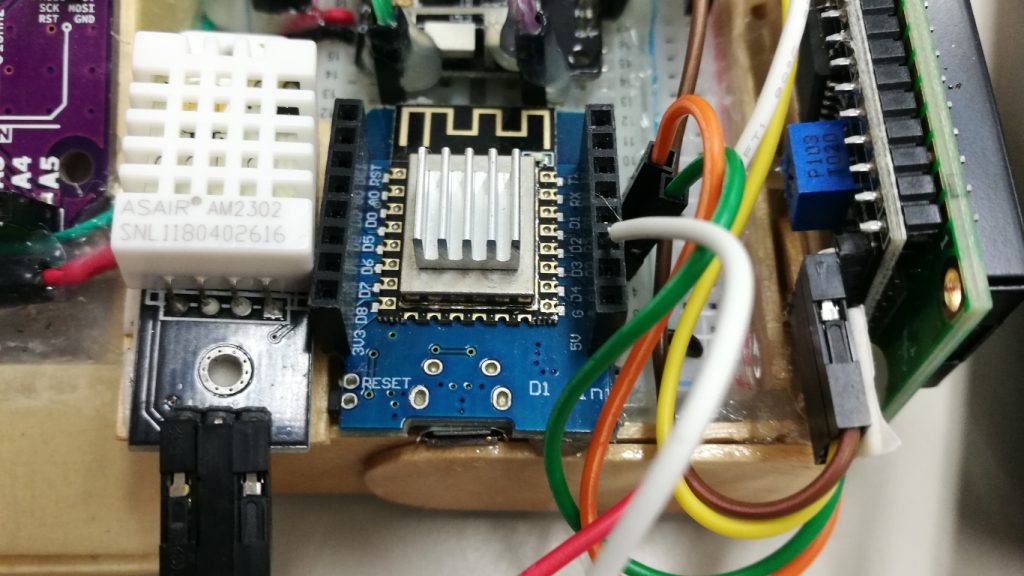
Library
To use the library for DHT22/DHT11 sensor module, the dht library must be called with the command
When running the library, the sensor object must be created by defining the connection pins as follows:
pin = machine.Pin( pin_number )
object = dht.DHT11( pin )or
pin = machine.Pin( pin_number )
object = dht.DHT22( pin )
The methods for reading and processing the data from the sensor are as follows.
object.measure()
The method for reading temperature after previously processing data from the sensor is as follows.
temperature = object.temperature()
The method for reading humidity data after previously processing data from the sensor is as follows.
humidity = object.humidity()
Example Code
Example code17-1 is reading data from the DHT22 sensor module and displaying it on the LCD display module with 16 characters per row, 2 rows. It is connected to the sensor module via GPIO2 and uses the I2C bus to connect to the LCD module.
For the case where the reader uses DHT11, the following line must be edited.
sensor = dht.DHT22( sensorPin )
to
sensor = dht.DHT11( sensorPin )
# code17-1
import sys
import gc
import os
import time
import dht
import machine as mc
from kjlcd import LCD
sclPin = mc.Pin(5) #D1
sdaPin = mc.Pin(4) #D2
sensorPin = mc.Pin(2) #D4
i2c = mc.I2C(scl=sclPin,sda=sdaPin)
sensor= dht.DHT22(sensorPin)
sensor.measure()
tempValue = sensor.temperature()
humidValue = sensor.humidity()
lcd_addr= const(0x27)
lcd = LCD(i2c,lcd_addr)
lcd.goto(0,0)
lcd.puts("T:{}C H:{}%".format(tempValue, humidValue))
lcd.goto(0,1)
lcd.puts("(C)2020,JarutEx")
Example code17-2 is an improvement of code17-1 to cycle every 2 seconds and find the lowest and highest temperature and humidity values to display on the display module, as shown in Figure 8.
# code17-2
import sys
import gc
import os
import time
import dht
import machine as mc
from kjlcd import LCD
sclPin = mc.Pin(5) #D1
sdaPin = mc.Pin(4) #D2
dht22Pin = mc.Pin(2) #D4
i2c = mc.I2C(scl=sclPin,sda=sdaPin)
dht22 = dht.DHT22(dht22Pin)
lcd_addr= const(0x27)
lcd = LCD(i2c,lcd_addr)
tMin = 99.0
tMax = 0.0
hMin = 99.9
hMax = 0.0
while True:
dht22.measure()
tempValue = dht22.temperature()
humidValue = dht22.humidity()
if (tMin > tempValue):
tMin = tempValue
if (tMax < tempValue):
tMax = tempValue
if (hMin > humidValue):
hMin = humidValue
if (hMax < humidValue):
hMax = humidValue
lcd.goto(0,0)
lcd.puts("T{} {}-{}".format(tempValue,tMin,tMax))
lcd.goto(0,1)
lcd.puts("H{} {}-{}".format(humidValue,hMin,hMax))
time.sleep_ms(1900)

Example code17-3 is using example code17-2 to find the average value from tMin/tMax, hMin/hMax and displaying the average value of temperature and humidity through the LCD module as shown in Figure 9. In this example, we added the method for controlling the display of decimal numbers uses a 4-character space and gives 1 decimal within those 4 characters. Therefore, the lcd.puts( ) command uses a format different from the previous example:
lcd.puts(“T-Average: {:4.1}”.format( tAverage ))
# code17-3
import sys
import gc
import os
import time
import dht
import machine as mc
from kjlcd import LCD
sclPin = mc.Pin(5) #D1
sdaPin = mc.Pin(4) #D2
dht22Pin = mc.Pin(2) #D4
i2c = mc.I2C(scl=sclPin,sda=sdaPin)
dht22 = dht.DHT22(dht22Pin)
lcd_addr= const(0x27)
lcd = LCD(i2c,lcd_addr)
tMin = 99.0
tMax = 0.0
hMin = 99.9
hMax = 0.0
while True:
dht22.measure()
tempValue = dht22.temperature()
humidValue = dht22.humidity()
if (tMin > tempValue):
tMin = tempValue
if (tMax < tempValue):
tMax = tempValue
if (hMin > humidValue):
hMin = humidValue
if (hMax < humidValue):
hMax = humidValue
tAverage = tMin + ((tMax-tMin)/2.0)
hAverage = hMin + ((hMax-hMin)/2.0)
lcd.goto(0,0)
lcd.puts("T-Average: {:4.1f}C ".format(tAverage))
lcd.goto(0,1)
lcd.puts("H-Average: {:4.1f}% ".format(hAverage))
time.sleep_ms(1900)
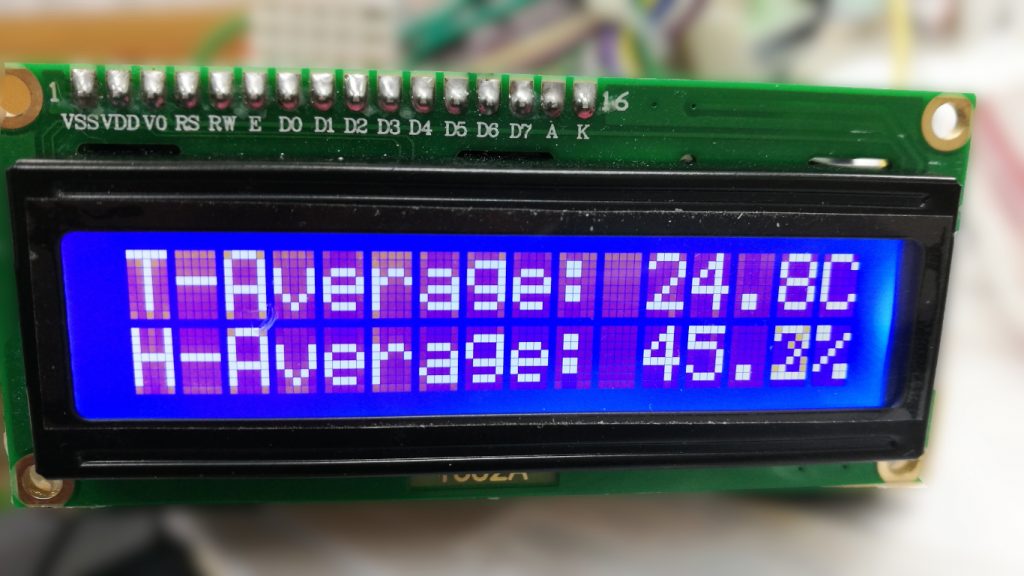
Conclusion
จากบทความนี้ผู้อ่านสามารถเขียนโปรแกรมภาษาไพธอนเพื่ออ่านค่าอุณหภูมิและความชื้นจากโมดูลเซ็น DHT22 หรือ DHT11 เพื่อแสดงผลที่โมดูลแอลซีดีตัวอักษรขนาด 2 แถว แะวละ 16 ตัวอักษร ซึ่งมีความแตกต่างกันในเรื่องของคุณสมบัติ และที่ต้องพึงระวังในการนำไปใช้งาน คือ ความเร็วในการทำงานของโมดูลเซ็นเซอร์ทั้ง 2 นี้มีความแตกต่างกัน ทำให้การวนรอบเพื่ออ่านค่าต้องสัมพันธ์กับการทำงานของเซ็นเซอร์ด้วย ดังนั้น เมื่อเลือกใช้โมดูลเซ็นเซอร์ DHT22 การวนรอบจะต้องไม่เร็วกว่า 2 มิลลิวินาที และสำหรับ DHT11 จะต้องไม่เร็วกว่า 1 มิลลิวินาที
From this article, the reader can write a Python program to read temperature and humidity values from the DHT22 or DHT11 sensor module to display on the LCD module with 2 rows of 16 characters each, which are different features and what you have to be careful is that the operating speeds of these 2 sensor modules are different. The cycle to read must be related to the operation of the sensor. Therefore, when using the DHT22 sensor module, the cycle cannot be faster than 2 ms and for the DHT11 it cannot be faster than 1 ms.
Finally, Happy Coding
References
(C) 2020, By Jarut Busarathud and Danai Jedsadathitikul
Updated 2024-0404