From reading articles on how to use board STM32L432 ADC and articles about STM32F103x connecting to ST7735S, it’s time to take STM32F103CBT6 or Blue-Pill/Black-Pill board. Let’s write a program to use ADC to display on a TFT screen. The example of the result of this article is as shown in Figure 1, which is reading from Pin PA0 that has been connected to Pin Analog signal from Board LDR in Figure 2 to Displayed on a TFT display.
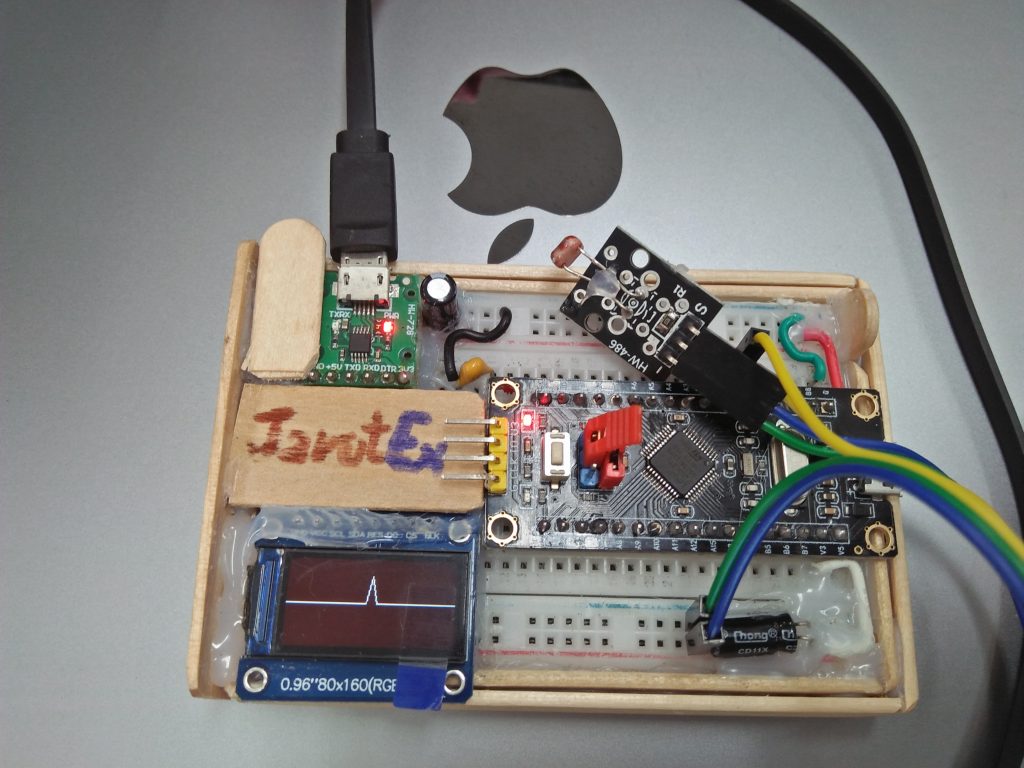
Equipment
- STM32F103CBT6 such as Blue-Pill, Black-Pill or ET-STM32F103
- LDR module as Figure 2
- The TFT screen module that uses the control chip ST7735s, in this article is RETAB type. The use of BLUETAB can be read from the article.
- USB/RS232 converter to use as part of the chip program or use ST-Link instead.
- Software
- Arduino IDE
- stm23duino
- TFT_eSPI library

Connection
The STM32F103CBT6 is connected to the TFT-LCD ST7735s as described in the ST7735s article. The connection to the LDR module is as follows.
- pin – of LDR connected to GND of STM32F103CBT6
- pin + of LDR connected to 3V3 of STM32F103CBT6
- pin S of LDR connected to PA0 of STM32F103CBT6
Example Code
The sample program contains an example of reading a value from an LDR via port PA0 and displaying it as text by drawing a straight line graph. There is working code as follows:
Display text
An example of this is to display a value read from an LDR by displaying text in small letters for 3 seconds, then in larger characters for 3 seconds as in Figures 3 and 4 after that, the cycle continues.
// micGraph.ino
// JarutEx
#include <TFT_eSPI.h> // Graphics and font library for ST7735 driver chip
#include <SPI.h>
TFT_eSPI tft = TFT_eSPI(); // Invoke library, pins defined in User_Setup.h
#define textColor TFT_WHITE
#define textBgColor 0x3861
#define pinMic PA0
#define ADC_BITS 12
String msg;
uint16_t dValue;
void setup() {
analogReadResolution(ADC_BITS);
tft.init();
tft.setRotation(1);
tft.writecommand(ST7735_MADCTL);
tft.writedata(TFT_MAD_MV | TFT_MAD_COLOR_ORDER );
tft.fillScreen(textBgColor);
}
void loop() {
dValue = analogRead( pinMic );
msg = "ADC value=";
msg += dValue;
tft.fillScreen( textBgColor );
tft.setTextColor( textColor );
tft.drawString( msg, 8 , 40, 1 ); // Font No.1
delay(3000);
tft.fillScreen( textBgColor );
tft.setTextColor( textColor );
tft.drawString( msg, 8 , 40, 2 ); // Font No.2
delay(3000);
}

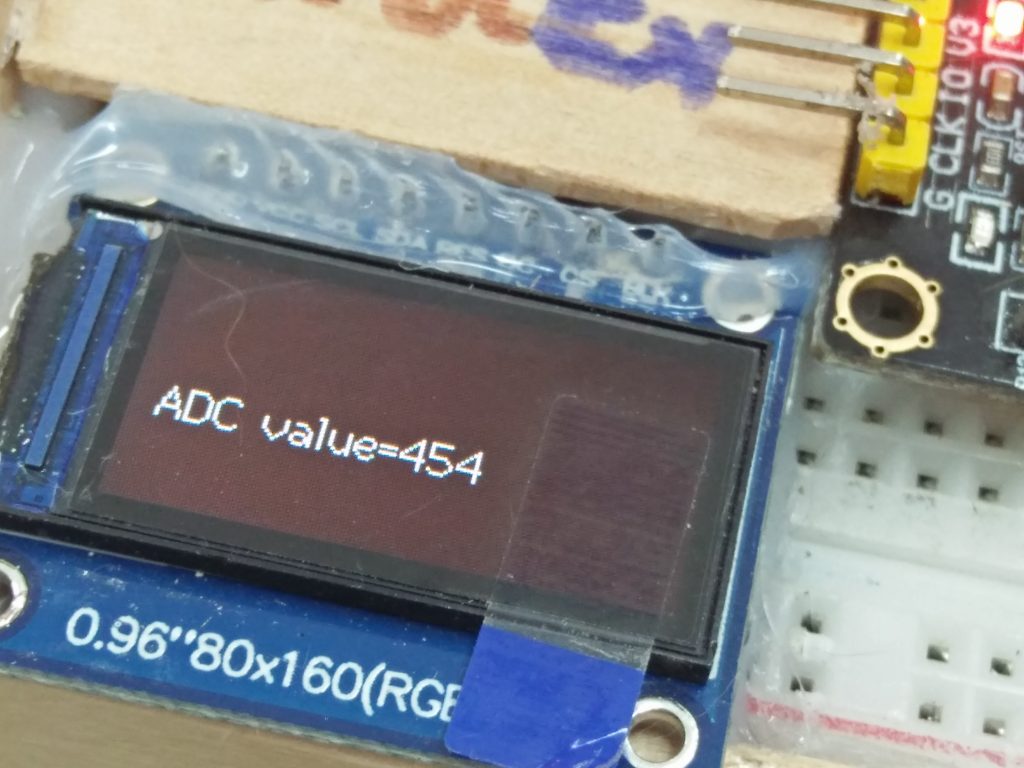
Display linear graph
This example changes the display from text to a straight line graph. The information is displayed in the location area (76,0)-(84,79) by converting the dValue value read from PA0 to a value in the range 0 to 79 for use as a value in the Y-axis by storing it in a variable named yValue, as shown in Figure 1. The program is as follows.
// micGraph.ino
// JarutEx
#include <TFT_eSPI.h> // Graphics and font library for ST7735 driver chip
#include <SPI.h>
TFT_eSPI tft = TFT_eSPI(); // Invoke library, pins defined in User_Setup.h
#define textColor TFT_WHITE
#define textBgColor 0x3861
#define pinMic PA0
#define ADC_BITS 12
uint16_t dValue;
uint16_t yValue = 0;
void setup() {
Serial.begin(115200);
analogReadResolution(ADC_BITS);
tft.init();
tft.setRotation(1);
tft.writecommand(ST7735_MADCTL);
tft.writedata(TFT_MAD_MV | TFT_MAD_COLOR_ORDER );
drawScr();
Serial.println("Started");
}
void drawScr() {
tft.fillScreen(textBgColor);
tft.drawLine(0, 40, 75, 40, textColor);
tft.drawLine(85, 40, 159, 40, textColor);
}
void loop() {
// อ่านค่า
dValue = analogRead( pinMic );
// ลบเส้นเดิม
tft.drawLine(75, 40, 80, yValue, textBgColor);
tft.drawLine(80, yValue, 85, 40, textBgColor);
// แปลง 0..4095 เป็น 0..79
yValue = (uint16_t)(((float)dValue / 4095.0) * 79.0);
// วาดเส้นใหม่
tft.drawLine(75, 40, 80, yValue, textColor);
tft.drawLine(80, yValue, 85, 40, textColor);
}
Conclusion
From this article, the STM32F103’s 12-bit resolution ADC is sensitive enough to input data for display on a TFT display, and readers will find the graphs barely updated (Due to ignoring the display rate) and will find that we have drawn the original graph instead of deleting the entire screen to save time in microcontroller operation and TFT operation. In addition, we can improve the code by importing the data in an array before displaying it to make it look like a beautiful continuous graph or adjusting the import rate to be the appropriate value, etc. Finally, have fun with programming.
(C) 2020-2022, By Jarut Busarathid and Danai Jedsadathitikul
Updated 2022-02-12