บThe ulab EP 5 discusses the numerical submodule used to calculate the minimum, maximum, sum, mean, and standard deviation. Enable convenience for working in calculating preliminary statistics. The article describes the functionality of the numerical submodule with an example program to illustrate how it works.
numerical
The numerical submodule provides the following operations with ndarray data.
- result = ulab.numerical.flip(M, axis=0) Reverse the position of the array by axis:
axis = 0 Reverse on the horizontal axis
axis = 1 Reverse on the vertical axis - ulab.numerical.roll(M, shift, axis=0) Shifts the data according to the number provides (positive values move left or down, negative values shift right or up) along with the defined axis as follows:
axis = 0 move horizontal axis
axis = 1 move vertical axis - ulab.numerical.sort(M, axis=0) Sorts the data in an array according to a given axis.
- result = ulab.numerical.argsott(M,axis=0) Creates an array of results from the order in which the data is arranged from array M.
There are also functions for use with 1D arrays:
- result = ulab.numerical.min( M ) Find the smallest value in M.
- result = ulab.numerical.max( M ) Find the largest value in M.
- result = ulab.numerical.argmin( M ) Finds the smallest value in M and returns the found position.
- result = ulab.numerical.argmax( M ) Finds the largest value in M and returns the found position.
- result = ulab.numerical.sum( M ) find the sum of M
- result = ulab.numerical.mean( M ) find the mean of M
- result = ulab.numerical.std( M ) Find the standard deviation of M.
Example Code 1
The example code18-11 is an example of the usage of an array inverting function, row shift and the sorting of rows in both 1D and 2D, which the result is shown in Figure 1.
# code18-11
import ulab as np
import time
import esp32
data1d = np.array([1,3,5,7,9],dtype=np.float)
data2d = np.array([[3,5,7],[9,11,13],[15,17,19]], dtype=np.float)
print("Original 1D ..... : {}".format(data1d))
print("Original 2D ..... : {}".format(data2d))
print("1D flip ......... : {}".format(np.numerical.flip(data1d)))
np.numerical.roll(data1d,-1)
print("1D left ......... : {}".format(data1d))
np.numerical.roll(data1d,1)
print("1D right ........ : {}".format(data1d))
print("2D H-flip ....... : {}".format(np.numerical.flip(data2d,axis=0)))
print("2D V-flip ....... : {}".format(np.numerical.flip(data2d,axis=1)))
np.numerical.roll(data2d,-1, axis=0)
print("2D H-left ....... : {}".format(data2d))
np.numerical.roll(data2d, 1, axis=0)
print("2D H-right ...... : {}".format(data2d))
np.numerical.roll(data2d,-1, axis=1)
print("2D V-up ......... : {}".format(data2d))
np.numerical.roll(data2d, 1, axis=1)
print("2D V-down ....... : {}".format(data2d))
np.numerical.sort(data1d)
print("Sorted 1D ....... : {}".format(data1d))
np.numerical.roll(data2d,1,axis=1)
np.numerical.sort(data2d,axis=0)
print("Sorted 2D-H ..... : {}".format(data2d))
np.numerical.roll(data2d,-2,axis=0)
np.numerical.sort(data2d,axis=1)
print("Sorted 2D-V ..... : {}".format(data2d))
np.numerical.roll(data2d,-2,axis=0)
np.numerical.roll(data2d,1,axis=1)
print("argsort 2D-H .... : {}".format(np.numerical.argsort(data2d,axis=0)))
print("argsort 2D-V .... : {}".format(np.numerical.argsort(data2d,axis=1)))
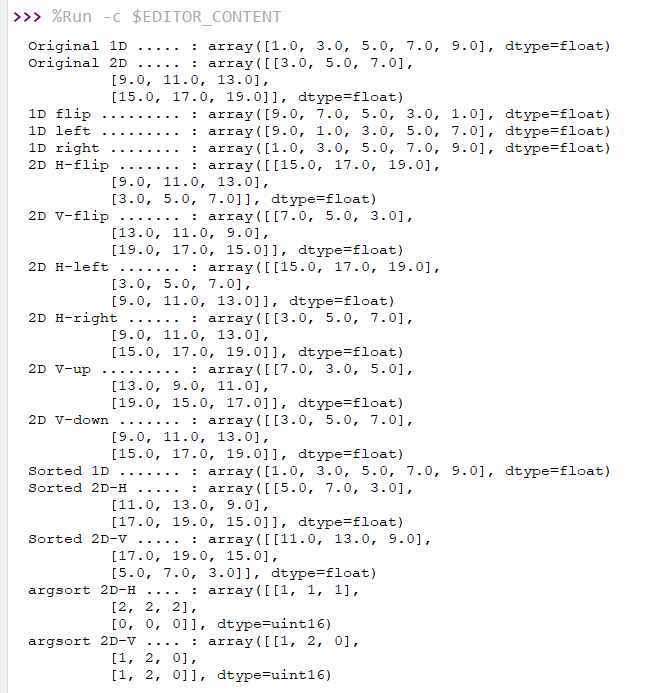
Example Code 2
The example code18-12 is a random sampling of 128 data using the quotient of the running time divided by the product of the ESP32’s temperature multiplied by the magnetic sensing value of the ESP32’s sensor, then the randomized values, minimum, maximum, sum, mean, and standard deviation are shown in Figure 2.
# code18-12
import ulab as np
import time
import esp32
def rndData(n):
data = np.array([0]*n,dtype=np.float)
for i in range(len(data)):
data[i] = time.ticks_ms()/(esp32.raw_temperature()*esp32.hall_sensor())
return data
data = rndData(128)
print("data ..... {}".format(data))
print("min ...... {} at {}".format(np.numerical.min(data),np.numerical.argmin(data)))
print("max ...... {} at {}".format(np.numerical.max(data),np.numerical.argmax(data)))
print("sum ...... {}".format(np.numerical.sum(data)))
print("average .. {}".format(np.numerical.mean(data)))
print("std ...... {}".format(np.numerical.std(data)))

Conclusion
From this chapter, Programmers can perform operations on arrays to reverse, shift, sort, and process 1D array values for minimum, maximum, sum, mean, and standard deviation.
Finally, have fun with programming.
(C) 2020, By Jarut Busarathid and Danai Jedsadathitikul
Updated 2021-08-18