This article is a compiled article about time library usage related to the time delay of the ESP8266 along with an example of connecting to an NTP Server for synchronization.
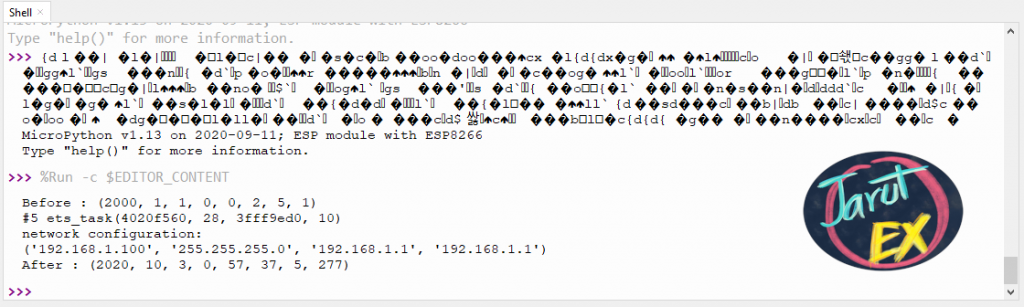
time
The time library is a library that deals with dates and times. This makes it possible to use the ESP8266 to store the date and time for various settings such as create a music alarm every 10th date or turning off the lights at 7.00 am, etc. The following command must be included before using the time library.
import time
To read the current time from ESP8266, use the following command format.
result = time.localtime( )
The results were stored in the form of tuple data of 8 members, each of which stores the data differently as follows
result[0] store year
result[1] store month, value from 1 to 12
result[2] store date of month, value from 1 to 31
result[3] store hour, value from 0 to 23
result[4] store minute, value from 0 to 59
result[5] store second, value from 0 to 59
result[6] store day, value from 0 to 6
result[7] store date of year, value from 1 to 366
For the case of setting the time to store in the ESP8266, the data storage format must be as follows.
(year, month, mday, hour, minute, second, weekday, yearday)
The command for setting the date and time has the following format:
time.mktime( dateTimeVar )
or
time.mktime( ( year, month, date, day, hour, minute, second, millisec ) )
For delays in seconds, milliseconds and microseconds can be operated with the following command.
time.sleep(seconds)
time.sleep_ms(millisec)
time.sleep_us(microsec)
An example of using the command to delay 3 seconds can be written as follows.
time.sleep(3)
or
time.sleep_ms( 3000 )
or
time.sleep_us( 30000000 )
Commands that read the current time in milliseconds, microseconds, or a CPU cycle are according to the format of the following command.
millisec = time.ticks_ms( )
microsec = time.ticks_us( )
cpuTime = time.ticks_cpu()
In addition, programmers can use the read time value to find the sum or difference with the following command.
timeSum = time.ticks_add( จำนวน, ค่าที่นำไปบวก )
timeDiff = time.ticks_diff( จำนวน1, จำนวน2 )
For example, to find the time in the next 2 seconds can be written as follows:
t0 = time.ticks_ms()
t1 = time.ticks_add( t0, 2000 )
An example of how to display a string “JarutEx” in next 2 seconds.
t0 = time.ticks_ms()
while (time.ticks_diff( time.ticks_ms( ), t0) < 2000):
pass
print("JarutEx")
1 hour = 60 minutes
1 minute = 60 seconds
1 second = 1,000 milliseconds
1 millisecond = 1,000 microseconds
Example of time syncing from NTP Server
Example of setting the time with the value read from a network time protocol service machine or NTP (Network Time Protocol) according to the program code5-1.
The principle of code5-1 is to rely on the RTC class as a link to the ntptime class, because when called ntptime.settime() the ntptime class reads the date and time from the internet. After that, set the date and time for the RTC class, which, when set to the RTC class result in the date and time information in both classes has been changed since they was designed to share a memory. Therefore, when settime() is performed, the system date and time are changed to world standard time.
# code5-1
import network as nw
import ntptime
from machine import RTC
import time
print("Before : {}".format(time.localtime()))
myESSID = "APName"
myPassword = "APPassword"
ifSTA = nw.WLAN(nw.STA_IF)
if (ifSTA.active() == False):
ifSTA.active(True)
ifSTA.connect(myESSID, myPassword)
while not ifSTA.isconnected():
pass
print("network configuration:\n{}".format(ifSTA.ifconfig()))
rtc = RTC()
ntptime.settime()
print("After : {}".format(time.localtime()))
ifSTA.active(False)
Conclusion
From this article you will find that the time library has four main functions: read/set date, delay time, read time, and perform sum or difference of time. This is important for programming that requires precision in terms of timing. Because Python is a high-level language that works as an interpreter leads to difficulty in control precision in the work. Hence, it needs to take a loop to read ticks and finding the difference to wait for the required time period. Additionally, we found that the time library uses the same memory space for storing date and time as the RTC library, thus setting the time from NTP resulting in the ESP8266 time changed to the world standard time.
The time from NTP is world standard time, so when applied to Thailand the programmers must always add the hour read plus 7 to change the time to Thailand time. If you want to change the year from A.D. to BE, the year must be added by 543.
Finally, the JarutEx team hopes this article will be helpful enough to readers as a guideline for further use. Have fun with programming.
If you want to discuss something, feel free to leave comments below.
(C) 2020, Danai Jedsadathitikul and Jarut Busarathid
updated 2020-10-05