An expression is a combination of operands and operators. An expression can be nested. However, programming principles differ from mathematics. For this reason, the conversion of an expression from a mathematical equation to an expression in a programming language requires a procedure to translate the correct sequence of calculations to prevent errors in calculations such as

y = 4/x2
It can be written in C++ as follows:
float x;
float y;
y = 4/x*x;
or
float x,y;
y = 4/(x*x);
which one is correct ?
Precedence
The order of precedence in action expressions is as follows.
- Do it in the deepest parentheses first.
- Do * or / or % from left to right.
- Do + or – from left to right.
Thus, if x = 4.5, calculating the equation y = 4/x2, the solution of 4/4.52 is 0.19753086419753085. But if you follow the expression written in C++ as in the previous example, you will get the following code and result:
Case 1
void setup() {
float x=4.5;
float y;
Serial.begin(115200);
y=4/x*x;
Serial.println(y);
}
void loop() {
}
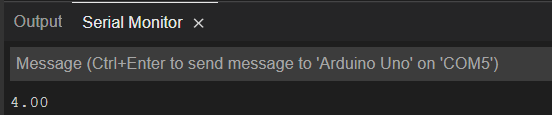
Case 2
void setup() {
float x=4.5;
float y;
Serial.begin(115200);
y=4/(x*x);
Serial.println(y);
}
void loop() {
}

From 2 cases, it was found that Case 2 gave the correct answer close to the calculation from a mathematical equation but Case 1 had a wrong calculation sequence, which is divided 4 by x, after which the result was multiplied with x because * and / have the same order of operation, they go from left to right, but in case 2, parentheses are required to calculate x*x or x2 first, then divide by 4 by the squared value and then calculate sequenced according to mathematical equations
In the case of mixed operators, the order of priority is as follows:
Precedence | Operators |
---|---|
1 | ( ) |
2 | * / % |
3 | + – |
4 | == != < > <= >= |
5 | ! |
6 | && |
7 | || |
Conclusion
From this article, we found that the sequence of operators affects the calculation process, so the reader must practice how to place the operators correctly. Alternatively, a separate computational approach may be used to simplify operations that require more memory to create temporary memory. However, there is no formula for writing an expression. Therefore, only practice, practice and practice. Finally, we hope you enjoy programming. Next time, it’s about programming the structure.
(C) 2020-2021, By Jarut Busarathid and Danai Jedsadathitikul
Updated 2021-09-27