Programming is an instruction for the processor to execute an instruction received. At a time, there is one instruction to process (in the case of non-parallel processing), once the instruction is completed, it saves the results of the commands in the working status register and prepares the next commands to be executed. This allows the operation to be performed individually from the first statement to the next, and the programmer can place the condition to separate the execution, for example, when a condition is true some instruction will be executed or any condition is not true, do not have to do or do something, etc. And finally, the function of the program can be repeated by the condition of iteration. For this reason, the basic programming principles consist of 3 types of work:
- Execute one command at a time from top to bottom.
- Able to add conditions of order processing.
- Able to repeat the desired command.

Repetition
The C++ or Python iteration principle uses conditional checks to make iterative decisions in a given group of statements. We selected while and do/while statements to describe the group of iterations because it is the basic form of programming in different languages.
While
If you want to repeat a section of instructions indefinitely as long as the condition is true, the while statement must be formatted as follows:
while ( condition ) {
things to do if condition is true
}
An example of writing a program to find the sum of integers in the range 1 to 100 can be written as follows:
// repetition: demo while
void setup() {
word sum=0;
word counter=0;
word endNumber = 100;
Serial.begin(115200);
Serial.println("---------- Begin of program ------------");
Serial.print("Before: sum = ");
Serial.println(sum);
while (counter <= endNumber) {
sum += counter;
counter += 1;
}
Serial.print("After: sum = ");
Serial.println(sum);
Serial.println("---------- End of Program ------------");
}
void loop() {
}
Result
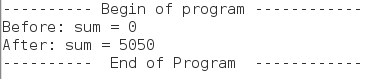
Do…While…
If you want to run one cycle first, then check the condition of the iteration. If the condition is true, it will revert to the previous statement again. There is a form of the command as follows:
do {
things to do
} while (condition);
An example of code to loop to find the sum of integers in the range 1 to 100 with do/while is as follows:
// repetition: demo do/while
void setup() {
word sum=0;
word counter=0;
word endNumber = 100;
Serial.begin(115200);
Serial.println("---------- Begin of program ------------");
Serial.print("Before: sum = ");
Serial.println(sum);
do {
sum += counter;
counter += 1;
} while (counter <= endNumber);
Serial.print("After: sum = ");
Serial.println(sum);
Serial.println("---------- End of Program ------------");
}
void loop() {
}
Conclusion
From this article, you are familiar with the syntax of the while and do/while iteration statements, which are the last execution patterns of structured programming, which are executed from top to bottom, can choose to act, and can repeat. This is a basic programming structure that has the same concept as other languages. We hope that it will be useful for further application or reading the codes in the future. Finally, have fun with programming. The basic commands of C++ language that are often used in Arduino are like this.
(C) 2020-2021, By Jarut Busarathid and Danai Jedsadathitikul
Updated 2021-09-30