This article is to learn how to use the Pin class which is a subclass in the machine class of Micropython for use with ESP8266 or ESP32 microcontrollers.
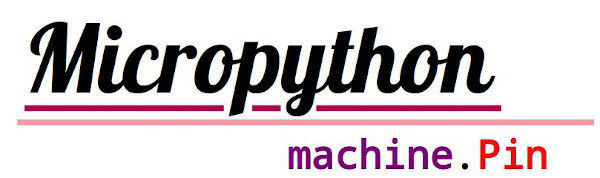
machine.Pin
Microcontroller’s pin or GPIO is responsible for inputting data or outputting data to connect devices or circuits outside the chip. Accessing these pins with Micropython must be done via the machine.Pin class with these steps.
- Import machine class
- Assign the pin
- Inputting or outputting the signal
Invocation of the Pin class can be done in two ways: through the machine class with the import machine and then using machine.Pin as the pin access point or specify to retrieve Pin from machine import Pin.
The command for defining pin functions has the following form of usage: where the work mode is set to machine.Pin.IN for data import and machine.Pin.OUT for data export. If you want to use a Pullup circuit or a connected circuit that uses a Pullup, specify machine.Pin.PULLUP. It can be assembled to create a pin object. In addition, if you want to initialize the pin from the start, you can set the mode to machine.Pin.OUT and let the value be either 0 or 1.
pin = machine.Pin( gpio, mode )
pin = machine.Pin( gpio, mode, machine.Pin.PULLUP )
pin = machine.Pin( gpio, machine.Pin.OUT, value=default )
The command to read a value and assign a value to a pin has the following syntax: on() is the same as value(1) and off() is the same as value( 0 ).
var = pin.value()
pin.on()
pin.off()
pin.value( value )
In addition to being used as part of the import and export of data, programmers can use the input pin as an interrupt actuator pin as well, as mentioned in the previous article.
GPIO’s properties
The functions of GPIO of microcontroller esp8266 and esp32 that must be known are as follows.
esp8266
- The pins that esp8266 provides for the designer or developer to use are 0,1,2,3,4,5, 12, 13, 14, 15 and 16.
- Pins 1 and 3 are Tx and Rx when used in serial communication (UART) on REPL systems.
- Pin 16 is used for wake up in power-saving mode by connecting to Rst pin.
- Pin A0 is used to operate a 10-bit ADC which supports 0-1VDC voltage.
- SPI communication (SPI0) works with communication speed up to 80MHz using the following pins.
- GPIO12 for performing MISO functions or importing data.
- GPIO13 for performing MOSI functions or exporting data.
- GPIO14 for performing SCK functions or generate a clock signal.
- I2C bus communication can be used on all pins because it works like Software I2C.
The esp8266 serial communication has the following pins:
pin | UART0 | UART1 |
---|---|---|
Tx | 1 | 2 |
Rx | 3 | 8 |
esp32
- esp32 has pins to be used as follows: Each pin can drive a maximum load of 40mA.
- Pins 0-19
- Pins 21-23
- Pins 25-27
- Pins 32-39 is a pin that supports ADC. It has a value range of 0 – 4095, but the voltage must be in the range of 0-1VDC. Normally, the board has a 0-3v3 voltage attenuation circuit to 0-1VDC, but it should be carefully checked before use.
- Pins 1 and 3 are REPL UART Tx and Rx, so if there is a serial communication connection, do not use these 2 pins to connect to an external circuit.
- Pin 6, 7, 8, 11, 16 and 17 are used to connect to the flash memory circuit of the microcontroller, so these pins should not be used with external circuits if the selected chip has an external flash ROM, especially the chip WROOM32, uses the pin to connect to the flash as follows.
- GPIO6 –> SCK/CLK
- GPIO7 –> SDO/SD0
- GPIO8 –> SDI/SD1
- GPIO9 –> SHD/SD2
- GPIO10 –> SWP/SD3
- GPIO11 –> CSC/CMD
- Pins for Capacitive Touch Sensors for use as wake up pins are:
- T0 –> GPIO4
- T1 –> GPIO0
- T2 –> GPIO2
- T3 –> GPIO15
- T4 –> GPIO13
- T5 –> GPIO12
- T6 –> GPIO14
- T7 –> GPIO17
- T8 –> GPIO33
- T9 –> GPIO32
- Pin 34-39 is a pin for acting as an input pin only, so it can’t work like machine.Pin.OUT.
- The pins for functioning DAC0 and DAC1 are GPIO25 and GPIO26.
- For some pins that machine.Pin.PULLUP and want to use it during power-saving mode or deepsleep set it to machine.PULLUP_HOLD
- These pins are specifically used for booting: GPIO 0, GPIO 2, and GPIO 4. The following 3 pins must have a mandatory state to boot.
- GPIO 1 must be HIGH
- GPIO 3 must be HIGH
- GPIO 5 must be HIGH during boot.
- GPIO 6 to GPIO11 connected with flash memory, should not be used
- GPIO 12 must be LOW during boot.
- GPIO 14 must be HIGH
- GPIO 15 must be HIGH during boot
- A pin that can perform ADC functions is a 12-bit ADC circuit.
- ADC1_CH0 –> GPIO 36
- ADC1_CH1 –> GPIO 37
- ADC1_CH2 –> GPIO 38
- ADC1_CH3 –> GPIO 39
- ADC1_CH4 –> GPIO 32
- ADC1_CH5 –> GPIO 33
- ADC1_CH6 –> GPIO 34
- ADC1_CH7 –> GPIO 35
- ADC2_CH0 –> GPIO 4
- ADC2_CH1 –> GPIO 0
- ADC2_CH2 –> GPIO 2
- ADC2_CH3 –> GPIO 15
- ADC2_CH4 –> GPIO 13
- ADC2_CH5 –> GPIO 12
- ADC2_CH6 –> GPIO 14
- ADC2_CH7 –> GPIO 27
- ADC2_CH8 –> GPIO 25
- ADC2_CH9 –> GPIO 26
For the pins connected to UART0, UART1 and UART2 are shown in the following table.
- UART0 used in the process of chip programming and during system reset/boot
- UART1 , on some boards it is used with SPI Flash.
- UART2 is a port that developers can continue to use.
Pin | UART0 | UART1 | UART2 |
---|---|---|---|
Tx/Send data | 1 | 10 | 17 |
Rx/Receive data | 3 | 9 | 16 |
The pins for connecting to SPI communication in the hardware mode of the ESP32 microcontroller are as follows.
Pin | HSPI (id=1) | VSPI (id=2) |
---|---|---|
SCK/generate clock signal | 14 | 18 |
MOSI/export data | 13 | 23 |
MISO/import data | 12 | 19 |
The pins for connecting to I2C communication in hardware mode are that the GPIO25 and GPIO26, they can be DAC, so if you choose to use a DAC, be careful when using the 2nd I2C (id=1).
Pin | I2C (id=0) | I2C (id=1) |
---|---|---|
SCL/Generate clock signal | 18 | 25 |
SDA/Send or receive data | 19 | 26 |
From this article, you will find that the pins of esp8266 and esp32 are enough for use. But if you look closely, you will find that many pins have limited functions. The remaining pins for connecting to the designed circuit are so few that sometimes we have chosen to use the I2C bus connection for connecting to the Arduino Uno/Mega2560 or STM32 board to expand the port. Finally, have fun with programming.
If you want to talk with us, feel free to leave comments below!!
References
- Micropython, “Quick reference for ESP8266”
- Micropython, “Quick reference for ESP32”
- Micropython, “class Pin – control I/O pins”
(C) 2020-2021, By Jarut Busarathid and Danai Jedsadathitikul
Updated 2021-11-02