This article is an application of the ESP-01s module connected to the ESP-01/01s Relay v4.0 as shown in Figure 1 to enable the relay to work. The example program is to turn the relay on and off via a web browser, which has been mentioned in some WebServer articles, but in addition to catching URLs for arguments to work.
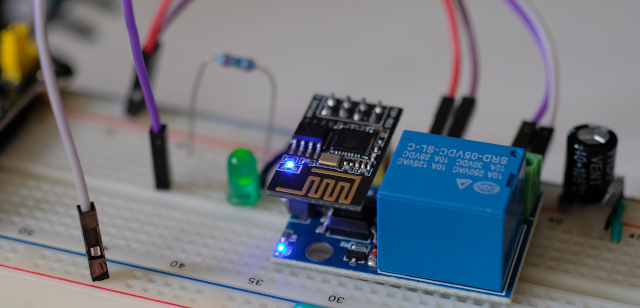
ESP-01/01s Relay
The ESP-01/01s Relay v4.0 module as shown in Figures 2 and 3 is a module designed for use with ESP-01 or ESP-01s. On the board, there is a connector for the ESP-01/01s module as shown in Figure 4 and the example of installing the board ESP-01s in Figure 5.
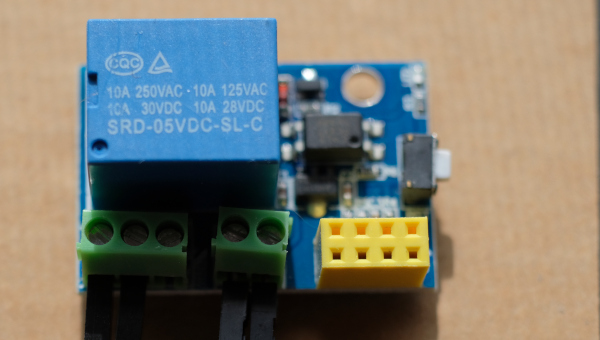
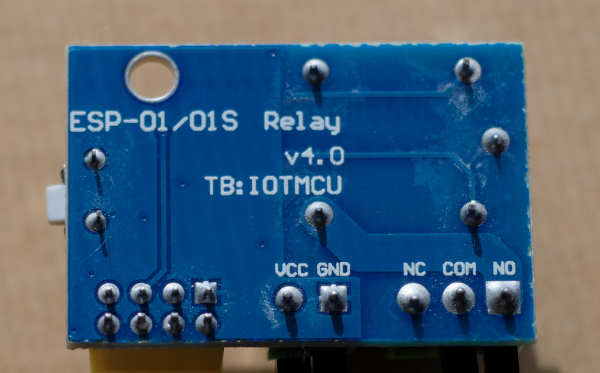

On the board there is a voltage converter from 5vdc to 3V3 with a reset button, making it convenient to use because the 5VDC (3.7-12vdc) voltage converter can be converted to 3V3 to make it possible to use the ESP-01 module or ESP-01s easier. The 3-channel connector header on the relay side is used to drive external loads in either DC or AC voltage.

The main features of the module are as follows.
- Compatible with both ESP-01 and ESP-01s boards.
- The installed relay can be used with DC 220V and drive current up to 10A.
- The relay connection has 3 terminals for NC, NO and COM.
- Operates at input voltage 3.7VDC to 12VDC.
- Requires more than 250mA of current, but by using the LD1117 which supports a maximum load of 800mA, it is sufficient for use.
The circuit diagram of the ESP-01/01s Relay v4.0 is shown in Figure 6. It is found that the pin for driving the relay is GPIO0 and uses LD1117 as a voltage converter.

From https://github.com/IOT-MCU/ESP-01S-Relay-v4.0/blob/master/ESP-01S%20Relay%20v4.0.pdf
Example Code
The first example program is to test the relay module by sending HIGH and LOW alternating every 10 seconds.
// Relay 5V
#define relayPin 0
#define ledPin 2
void setup() {
pinMode( relayPin, OUTPUT );
pinMode( ledPin, OUTPUT );
}
void loop() {
digitalWrite( ledPin, HIGH );
digitalWrite( relayPin, HIGH );
delay(10000);
digitalWrite( ledPin, LOW );
digitalWrite( relayPin, LOW );
delay(10000);
}
In this lab test, the load is driven by an LED lamp as shown in Figure 7 instead of an AC lamp (to protect the author of the article).
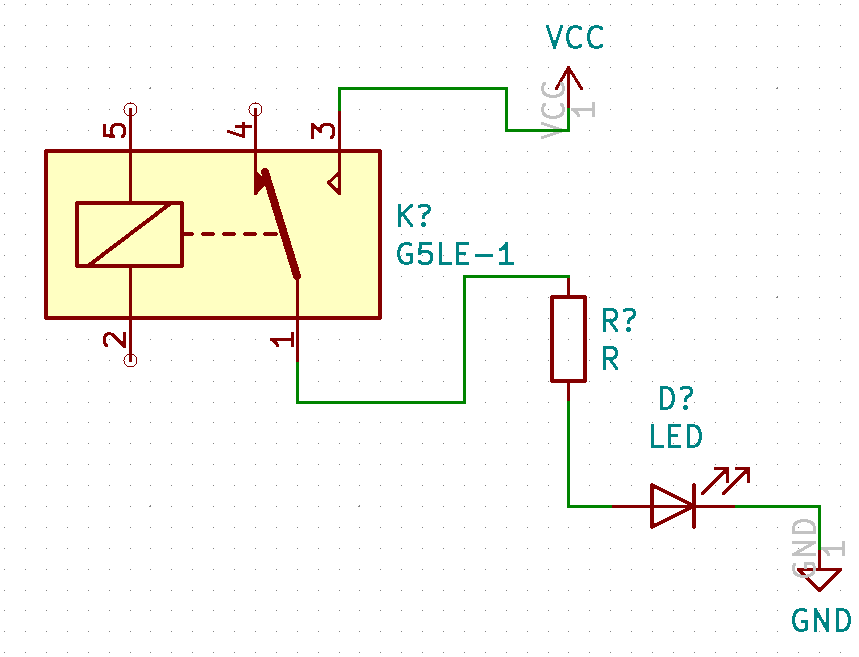
From the previous article, how the web service works are to report to the user. But in the case of importing data from users, it relies on web components that are imported from forms and send parameters in the form of HTTP GET Requests, for example:
<form action='/resource' id='#form'>
</form>
<button type="submit" form="รหัสชองฟอร์ม" value="Submit">ข้อความบนปุ่ม</button>
From the form above, when writing the following HTML language, it can be seen that if the user presses a button, the web will be called with /abc? where /abc is what we use to define server.on(“/abc”, responsive function )
<form action='/abc' id='controlForm'>
</form>
<button type="submit" form="controlForm" value="Submit">Press</button>
The relay control program code through the web page is as follows.
// control Relay
#include <ESP8266WiFi.h>
#include <ESP8266WebServer.h>
#define AP_NAME "JarutEx"
#define AP_PASSWD "123456789"
#define relayPin 0
#define ledPin 2
IPAddress myIP(192, 168, 4, 1);
IPAddress gwIP(192, 168, 4, 10);
IPAddress subnet(255, 255, 255, 0);
ESP8266WebServer server(80);
void setup() {
pinMode( relayPin, OUTPUT );
pinMode( ledPin, OUTPUT );
if (WiFi.softAPConfig( myIP, gwIP, subnet )) {
if (WiFi.softAP(AP_NAME, AP_PASSWD, 8, false, 5)) {
digitalWrite( ledPin, HIGH );
} else {
digitalWrite( ledPin, LOW );
while (true);
}
} else {
while (true) {
digitalWrite( ledPin, LOW );
delay(100);
digitalWrite( ledPin, HIGH );
delay(100);
}
}
server.on("/", htmlPage);
server.on("/turnOn", doTurnOn);
server.on("/turnOff", doTurnOff);
server.begin();
}
int relayStatus = 0; // 0 = off, 1 = on
void doTurnOn() {
relayStatus = 1;
digitalWrite( relayPin, LOW );
htmlPage();
}
void doTurnOff() {
relayStatus = 0;
digitalWrite( relayPin, HIGH );
htmlPage();
}
void htmlPage() {
String html;
html.reserve(2048); // prevent ram fragmentation
html = F(
"<!DOCTYPE HTML>"
"<html><head>"
"<meta name='viewport' content='width=device-width, initial-scale=1'>"
"<style>"
"html {font-family: Arial; display: inline-block; text-align: center;}"
"h1 {font-size: 3.0rem;}"
"p {font-size: 3.0rem;}"
"body {max-width: 800px; margin:0px auto; padding-bottom: 16px;}"
".switch {position: relative; display: inline-block; width: 120px; height: 68px} "
".switch input {display: none}"
".slider {position: absolute; top: 0; left: 0; right: 0; bottom: 0; background-color: #ccc; border-radius: 34px}"
".slider:before {position: absolute; content: ''; height: 52px; width: 52px; left: 8px; bottom: 8px; background-color: #fff; -webkit-transition: .4s; transition: .4s; border-radius: 68px}"
"input:checked+.slider {background-color: #2196F3}"
"input: checked+.slider:before {-webkit-transform: translateX(52px); -ms-transform: translateX(52px); transform: translateX(52px)}"
".button {"
"background-color: #4CAF50; /* Green */"
"border: none;"
"color: white;"
"padding: 32px 32px;"
"text-align: center;"
"text-decoration: none;"
"display: inline-block;"
"font-size: 16px;"
"width: 10.0rem;"
"height: 10.0rem;"
"border-radius: 50%;"
"}"
".buttonOn {background-color: #f44336;}"
".buttonOff {background-color: #555555;}"
"</style>"
"</head><body>"
"<h1>ESP-01/01a Relay V4.0</h1>"
);
if (relayStatus == 0) {
html += F("<div><form action='/turnOn' id='controlRelay'>" // input type='button'>On/Off</input></div>");
"</form>"
"<button type='submit' class='button buttonOn' form='controlRelay' value=Submit'>Turn On</button>"
"</div>"
);
} else {
html += F("<div><form action='/turnOff' id='controlRelay'>" // input type='button'>On/Off</input></div>");
"</form>"
"<button class='button buttonOff' type='submit' form='controlRelay' value=Submit'>Turn Off</button>"
"</div>"
);
}
html += F("</body></html>\r\n");
server.send(200, "text/html", html);
}
void loop() {
server.handleClient();
}
Figure 8 is an example of when HIGH is sent to the relay and Figure 9 is an example when LOW is sent which results in an open circuit or the light is off and LED is on (Please check the circuit of the relay side well because they can be connected and work vice versa). Figures 10 and 11 are examples of usage from a web browser.

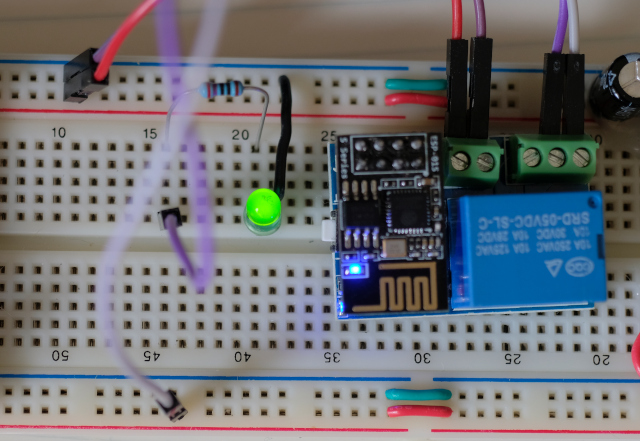
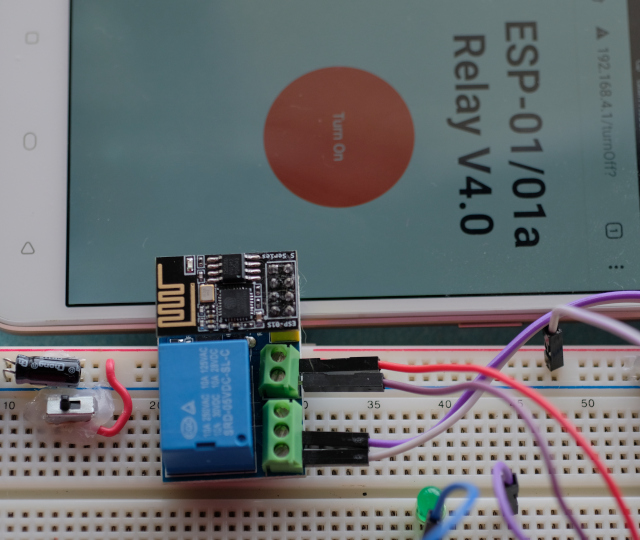
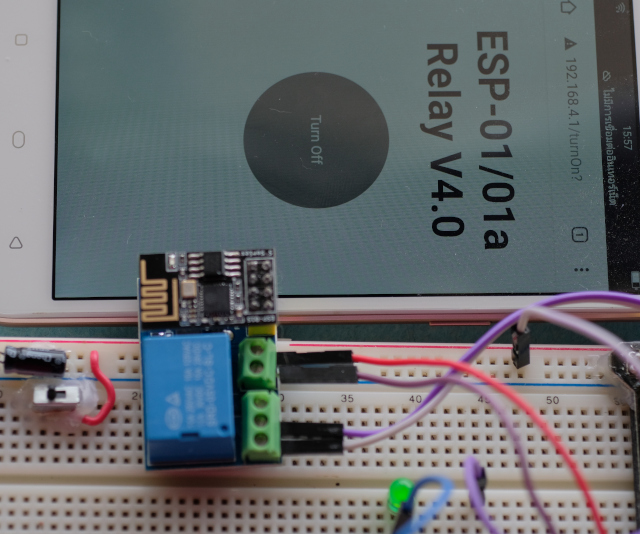
Conclusion
From this article, you will find that the ESP-01/01s Relay Module is very convenient to install and use because on the board there is a power converter from 3.7-12VDC to 3V3 to feed the ESP-01s board and use 5VDC from the Power Bank got a good result as well. But if you want to turn off the alternating current, we urge the subjects to be careful as they are dangerous. And the important question is how to make a beautiful web page? Finally, have fun with programming.
If you want to talk with us, feel free to leave comments below!!
References
- IOT MCU github : ESP-01/01s Relay V4.0
- IOT MCU Datasheet : ESP-01/01s Relay V4.0 Document
- ST : LD1117
- Random Nerd Tutorial : ESP8266 NodeMCU Relay Module – Control AC Appliances (Web Server)
- Random Nerd Tutorial : Input Data on HTML From ESP32/ESP8266 Web Server using Arduino IDE
(C) 2021, By Jarut Busarathid and Danai Jedsadathitikul
Updated 2021-11-13