This article discusses using a Ticker with an ESP8266 microcontroller to generate clock interrupts instead of delaying with delay(), the amount of time spent by looping with delay() is used to do other things and create efficiency for the developed software.
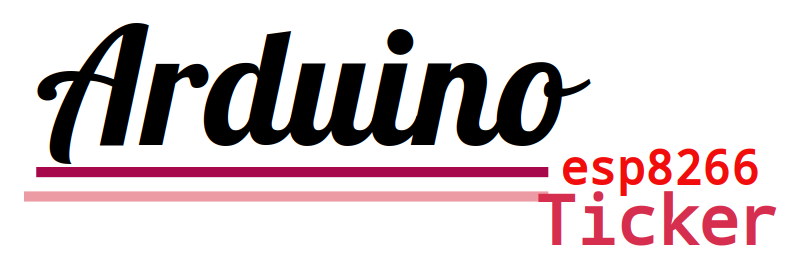
การใช้งาน Ticker
Ticker is a library of esp8266 developed since 2014 by Ivan Grokhotkov that enables the Arduino framework to access using os_timer to set a timer to call a given callback function after a certain amount of time has passed. By using it, you need to run the header file Ticker.h as follows:
#include <Ticker.h>
Once the ticker header file is imported, the next step is to create a timer object created from the Ticker class:
Ticker obj;
The use of a timer object must define the duration of the timer in seconds (using decimal numeric data) and specify the function to be called after a specified period, in the following format:
obj.attach( sec, callback)
If you want to set the value in milliseconds, change the function to attach_ms() instead of attach() as in the following format.
obj.attach_ms( mil, callback )
For a single call to a given function, it can be changed to use once() or once_ms() as follows:
obj.once( sec, callback )
obj.once_ms( mil, callback )
To check the running state of the created timer object, use the active() statement to check its status: the answer is true or false
status = obj.active()
In case attach() or attach_ms() is used and want to stop the recursion, you can un-append the function to the timer with detach() :
obj.detach()
Additionally, if you want to create a parameter to a response function, you must use std::bind(), such as the following example.
void func( int id ) {
Serial.println( id );
}
...
obj.attach_ms(500, std::bind( func, 10 ));
...
Example Code
From the sample code below, we use the delay method of the delay() command to order the LEDs on the NodeMCU to turn on and off alternately every 500ms (approximately).
void setup() {
pinMode(LED_BUILTIN, OUTPUT);
}
void loop() {
digitalWrite(LED_BUILTIN, LOW);
delay(500);
digitalWrite(LED_BUILTIN, HIGH);
delay(500);
}
When recoding using Ticker, there are three main writing steps: creating a Ticker scheduling object, creating a responsive function and appending a function to a timer object as the following code.
#include <Ticker.h>
Ticker tm500ms;
void myToggle() {
int state = digitalRead(LED_BUILTIN); // get the current state of GPIO1 pin
digitalWrite(LED_BUILTIN, !state); // set pin to the opposite state
}
void setup() {
pinMode(LED_BUILTIN, OUTPUT);
digitalWrite(LED_BUILTIN, LOW);
tm500ms.attach(0.5, myToggle);
}
void loop() {
}
When observing the working principle of both programs, it is found that the first program was turned on (or closed) and delayed by 500 milliseconds, after that, turned off (or turned on) and delayed another 500 milliseconds, so the actual elapsed time exceeded 1 second, but in the second program when using Ticker, it is triggered every 500 milliseconds, meaning that once the set time has elapsed the program will read the status of the LED_BUILTIN pin and invert the value to cause the lamp to switch on or off. Then end the work, and after 500 ms, it will switch on or off again. Therefore, writing in type 2 will result in 1 second or near 1 second will have 1 cycle on and off. For the remaining time we will be able to write programs to perform other tasks. Because when the set time is reached, it will stop the work in progress and return to respond to the on / off command. But in the first method it takes time to delay() .
Conclusion
From this article, you will find that Ticker supports the work of scheduling. It supports both setting a time to do it at any given time and can cancel the work later with a timer to run only once. When applied to applications in the form of setting the time for the microcontroller to collect values from DHT11 every 1 minute, it will be able to store a detailed temperature and humidity database every 1 minute as well, or apply it as a clock. All of which depend on experience and application. Finally, have fun with programming.
If you want to talk with us, feel free to leave comments below!!
Reference
- Github: esp8266/Arduino/Libraries/Ticker
(C) 2020-2021, By Jarut Busarathid and Danai Jedsadathitikul
Updated 2021-11-22