In this article, let’s understand and write a program to find the nth order from a list of data in Python, tested with Python and Micropython.
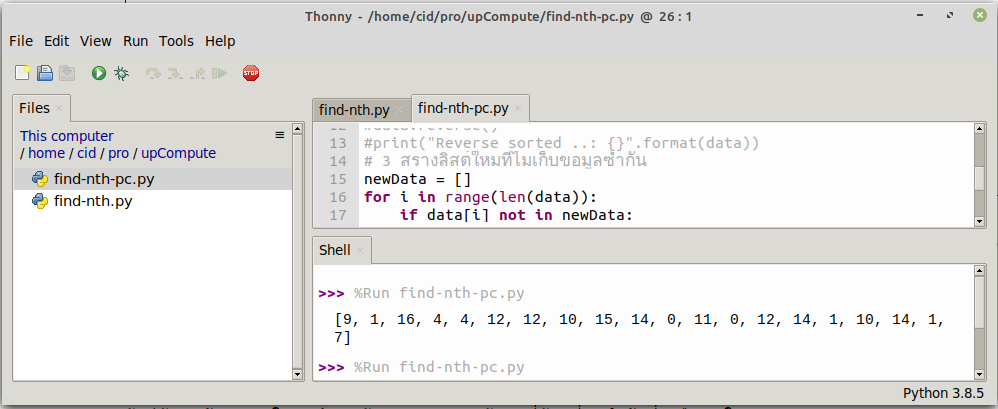
Problem
If there are numeric data in the list and want to know what is the nth smallest data value.
Concept
From the problem established, when considering, there is a list of minor problems that was found including:
- Is the stored data sorted? Because if sorted, it means that finding the largest and smallest values is just looking at the data in the desired order.
- If not sorted, how do we sort the data? and how to arrange to ascend.
- What if there are duplicates in the list?
- What if the sequence we want to see is out of the range of the sequence?
Data preparation
Out of these four items, the first thing you need to do is to collect the data in the list. In this experiment, random values were used instead of receiving inputs. We random 20 integer numbers. esp8266 and esp32, use the following code: an example of the random result is shown in Figure 1.
import random
# 1 random
data = []
for i in range(20):
data.append(random.getrandbits(4))
print(data
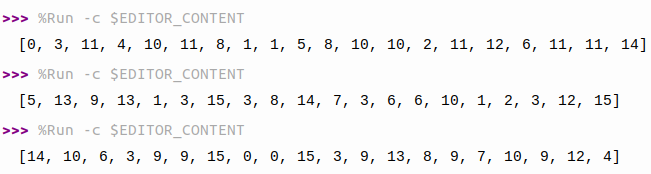
For Raspberry Pi or general PC, use the following code randomization and an example of random sampling is shown in Figure 2.
import random
# 1 random
data = []
for i in range(20):
data.append(random.randint(0,16))
print(data)
From the above code we can see that the random value is used as a randint() command to randomize the values from a to (b-1) according to the following usage patterns:
random_var = random.randint( a, b )

As an example of randomization, it is found that a list object of type data was created without initializing it. The format of creating list data is as follows.
list_obj = []
When a list-type object or list-type variable has been created successfully, Adding items to a list can be done by using the append() in the following format.
list_obj.append( data )
Sorting
The sorting method of a list structure in Python uses the sort() in the following format.
list_obj.sort()
From the example in Figure 3, we can see that sort() is sorted in ascending order. If you want it to be sorted in descending order, you need to reverse the order of the list items with the reverse() command as follows:
list_obj.reverse()
An example program to sort data from random is as follows and the sample result is as shown in Figure 3.
print("Raw data ........: {}".format(data))
data.sort()
print("Sorted ..........: {}".format(data))
data.reverse()
print("Reverse sorted ..: {}".format(data))

Create new list
From Figures 1, 2 and 3, it can be seen that the randomized data is likely to be duplicated. When it happened as in Figure 3, if wanting to know the smallest first, then there are 2 values, 0 in the order of 0 and 1. Therefore, when finding the answer with the following command, you will get the correct answer.
print(“1st = {}”.format(data[0]))
But if we look at the 2nd order with the following statement, the answer is still 0, which is the smallest value first.
print(“2nd = {}”.format(data[1]))
So, we’ll create a new, non-duplicating list by doing the following:
print("Raw data ........: {}".format(data))
data.sort()
print("Sorted ..........: {}".format(data))
newData = []
for i in range(len(data)):
if data[i] not in newData:
newData.append( data[i] )
print("New list ........: {}".format(newData))
As a result, the new list created may be the same size or less and the members within the list have no duplicate values as in Figure 4.

Checking or searching for items in a list to decide whether to add them to the list using in a statement, the answer is true or false as the following format
data in list_obj
So, if you want to check if this item is not in the list, change the writing style to the following:
data not in list)obj
Check range of data
Examining the data range to prevent sequencing greater or less than the range of list variables obtained in the previous step requires an understanding of the attributes of list-type variables. The first order is 0th and the last order is -1 and so on.
Therefore, the incoming value must not exceed the maximum size of the list variable, which is obtained by counting the number of members in a list with the len() as follows:
members = len( list)obj )
Therefore, the range of values used in the sequence is 1 to (number of members +1) and the value used to find n is 0 to (number of members).
Search and report
The result in Figure 5 is an example from the first lesser value. Searching for sequences beyond the available range and searching for the second data as shown in the following sample code.
n = -5
if (n in range(1, len(newData)+1)):
print(newData[n-1])
else:
print("{} : Out of range!".format(n))
n = 100
if (n in range(1, len(newData)+1)):
print(newData[n-1])
else:
print("{} : Out of range!".format(n))
n = 2
if (n in range(1, len(newData)+1)):
print(newData[n-1])
else:
print("{} : Out of range!".format(n))

Example Code
The idea of solving all the problems can be written as a program for esp8266 and esp32 as follows.
# how can we find nth from the list?
import random
# 1 random
data = []
for i in range(20):
data.append(random.getrandbits(4))
# 2 sort
print("Raw data ........: {}".format(data))
data.sort()
print("Sorted ..........: {}".format(data))
# 3 create new set
newData = []
for i in range(len(data)):
if data[i] not in newData:
newData.append( data[i] )
print("New list ........: {}".format(newData))
# 4-5 find
n = int(input("data?"))
if (n in range(1, len(newData)+1)):
print(newData[n-1])
else:
print("{} : Out of range!".format(n))
For Raspberry Pi or PC with Windows, macOS or Linux write as follows.
# how can we find nth from the list?
import random
# 1 random
data = []
for i in range(20):
data.append(random.randint(0,16))
# 2 sort
print("Raw data ........: {}".format(data))
data.sort()
print("Sorted ..........: {}".format(data))
# 3 create new set
newData = []
for i in range(len(data)):
if data[i] not in newData:
newData.append( data[i] )
print("New list ........: {}".format(newData))
# 4-5 find
n = int(input("data?"))
if (n in range(1, len(newData)+1)):
print(newData[n-1])
else:
print("{} : Out of range!".format(n))
From the example program, it is found that the part of receiving n from the user is written by input(), which has the following form:
imported = input(text)
Since the input value may not be an integer in some systems, convert the input to an integer with int() as follows:
int_data = int( data )
Conclusion
From this article, you will find that solving problems with Python is easy as it has a full library for users. But the solution is something that programmers have to think and explain programming clearly in order to be able to translate it into a set of instructions. Finally, have fun with programming.
If you want to talk with us, feel free to leave comments below!!
Reference
- Dalke Andrew and Hettinger Raymond. “Sorting HOW TO”
(C) 2020-2021, By Jarut Busarathid and Danai Jedsadathitikul
Updated 2021-11-06